GitLab is an open-source DevOps platform, delivered as a single application. This makes GitLab unique and creates a streamlined software workflow, unlocking your organization from the constraints of a pieced-together toolchain.
GitLab is essentially a web-based Git repository that gives you the ability to create free open and private repositories, with issue-following capabilities, and wikis. It is also a complete DevOps platform that enables your team to perform all their tasks in a project, starting from the project planning phase and source code management to monitoring and security. Most importantly, it gives teams the ability to collaborate and build good software.
One of the many perks of using GitLab’s API is that it gives you and your team the flexibility to perform many of the actions you would normally carry out using the user interface. Employing the use of the API allows you to automate the process and eliminate the need for any user interaction. There are two major ways to interact with the GitLab API:
- Rest API
- GraphQL API
GitLab provides a REST API for easy access using regular API requests. The GitLab API can also be accessed with GraphQL. Using GraphQL, you can make an API request for only what you need, and it’s versioned by default.
Authentication and Security
Most API requests that demand sensitive information and private repositories require authentication; others may only return publicly accessible data when authentication is not provided. When authentication is not required, the documentation for each endpoint specifies this. For example, the `/projects/:id`
endpoint does not require authentication.
However, the GitLab API provides several methods for authenticating users before allowing access to certain high-level actions. Some of the authentication methods include:
OAuth2 Tokens
OAuth2 tokens can be employed as a way to authenticate users via the GitLab API. The token can be passed as an `access_token`
parameter to the base URL or as an Authorization header.
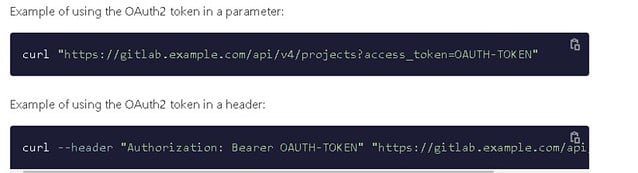
Personal/Project Access Tokens
In order to use personal access tokens, you can authenticate with the API by passing it in either the `private_token`
parameter or passing it as the `PRIVATE-TOKEN`
header. An example of how each would look is provided below.
Passing personal access tokens as a URL parameter:
curl "https://gitlab.example.com/api/v4/projects?private_token=<your_access_token>"
Passing personal access tokens as a header:
curl --header "PRIVATE-TOKEN: <your_access_token>" "https://gitlab.example.com/api/v4/projects"
It is important to note that it is also possible to use personal or project tokens with OAuth2 compliant headers. That would look something like this:
curl --header "Authorization: Bearer <your_access_token>" "https://gitlab.example.com/api/v4/projects"
Session Cookies
When you sign into the main GitLab web application, it sets a `_gitlab_session`
cookie. The API uses this cookie for authentication if it’s present. There is currently no way to generate a new session cookie using the GitLab API.
The major user of this authentication method is the web frontend of GitLab itself. The web frontend can use the API as the authenticated user to get a list of projects without explicitly passing an access token.
GitLab CI/CD Job Tokens
Using the GitLab API, GitLab generates a unique token and injects it as the `CI_JOB_TOKEN`
predefined variable whenever a pipeline job is about to run.
The token has the same permissions to access the API as the user that triggers the pipeline. Therefore, this user must be assigned to a role that has the required privileges.
The CI/CD job token is only valid for the lifetime of the pipeline task. You won’t be able to use the token after the job is completed.
Without a configuration, a job token can access project resources, but it could give additional unnecessary privileges. You can also use the job token to authenticate and clone a repository from a private project in a CI/CD job:
git clone https://gitlab-ci-token:${CI_JOB_TOKEN}@gitlab.example.com/<namespace>/<project>
SCIM API
GitLab also provides support for SCIM (System for Cross-Domain Identity Management), an open standard that allows user provisioning.
According to the GitLab docs, when SCIM is provisioned for a GitLab group, membership of that group is synchronized between GitLab and the identity provider.
With the support for SCIM, you can carry out actions such as creating and deactivating users. Also, identity providers such as Azure and Okta are supported. If you’re interested in learning to configure any of them, visit this page and learn how to get started.
Continuous Integration/Continuous Deployment (CI/CD)
CI/CD, or continuous integration and continuous delivery, is an industry-standard practice and guideline for managing teams as they work through the lifecycle of an application, all the way from the development phase to production, updates, and reintroduction of features.
Continuous integration is a programming philosophy and set of techniques that encourage development teams to make little changes and regularly check code into version control repositories. Because most current applications include writing code across several platforms and tools, the team requires a way to integrate and validate its modifications.
The technical objective of CI is to provide a standardized and automated process for developing, packaging, and testing applications. Teams are more inclined to commit code changes more frequently when the integration process is consistent, which leads to greater collaboration and improved software quality.
API Endpoints to Manage a Repo Lifecycle
The major activities for creating and maintaining a repository can be carried out using the GitLab API. Here’s an overview of a few of them. If you wish to follow along, you need to have a local GitLab install as well as jq installed.
export GITLAB_SERVER=http://localhost
export GITLAB_TOKEN=sR4NN_HsMxhHWgju17pn
export GITLAB_ROOTGROUP=my-programme
export GITLAB_SUBGROUP=backhub
export GITLAB_PROJECT=hello-world-project-01
export GITLAB_FEATURE=feature/my-feature-0
#creating a root group
rootGroupId=$(curl --header "PRIVATE-TOKEN: $GITLAB_TOKEN" "$GITLAB_SERVER/api/v4/groups?search=$GITLAB_ROOTGROUP" | jq '.[0]["id"]' )
if [ $rootGroupId == "null" ] then rootGroupId=$(curl -d "name=$GITLAB_ROOTGROUP&path=$GITLAB_ROOTGROUP&visibility=private&lfs_enabled=true&description=Root group" -X POST "$GITLAB_SERVER/api/v4/groups" -H "PRIVATE-TOKEN: $GITLAB_TOKEN" | jq '.["id"]') fiecho "Root group Id: $rootGroupId"
#creating a sub group
rootSubGroupId=$(curl --header "PRIVATE-TOKEN: $GITLAB_TOKEN" "$GITLAB_SERVER/api/v4/groups/$rootGroupId/subgroups?search=$GITLAB_SUBGROUP" | jq '.[0]["id"]' )
if [ $rootSubGroupId == "null" ]
then rootSubGroupId=$(curl -d "name=$GITLAB_SUBGROUP&path=$GITLAB_SUBGROUP&visibility=private&lfs_enabled=true&description=$GITLAB_SUBGROUP programme&parent_id=$rootGroupId" -X POST "$GITLAB_SERVER/api/v4/groups" -H "PRIVATE-TOKEN: $GITLAB_TOKEN" | jq '.["id"]')
fi
echo "Sub group Id: $rootSubGroupId"
#creating a project
projectId=$(curl "$GITLAB_SERVER/api/v4/groups/$rootSubGroupId/projects?search=$GITLAB_PROJECT" -H "PRIVATE-TOKEN: $GITLAB_TOKEN" | jq '.[0]["id"]' )
if [ $projectId == "null" ]
then projectId=projectId=$(curl -d "path=$GITLAB_PROJECT&namespace_id=$rootSubGroupId" -X POST "$GITLAB_SERVER/api/v4/projects" -H "PRIVATE-TOKEN: $GITLAB_TOKEN" | jq '.["id"]')
fi echo "Project Id: $projectId"
#cloning a Git project and generating a basic Java Maven structure
mvn archetype:generate -B -DarchetypeGroupId=org.apache.maven.archetypes -DarchetypeArtifactId=maven-archetype-quickstart -DarchetypeVersion=1.4 -DgroupId=edu.emmerson.gitlab -DartifactId=$GITLAB_PROJECT -Dversion=0.0.1
cd $GITLAB_PROJECT
git init
git remote add origin
git@localhost:$GITLAB_ROOTGROUP/$GITLAB_SUBGROUP/$GITLAB_PROJECT.git
git add .
git commit -m "Initial commit"
git push -u origin master
#Git branches initial structure as per Gitflow
projectId=$(curl "$GITLAB_SERVER/api/v4/groups/$rootSubGroupId/projects?search=$GITLAB_PROJECT" -H "PRIVATE-TOKEN: $GITLAB_TOKEN" | jq '.[0]["id"]' )
#create develop branch
curl -d "branch=develop&ref=master" -X POST "$GITLAB_SERVER/api/v4/projects/$projectId/repository/branches" -H "PRIVATE-TOKEN: $GITLAB_TOKEN" | jq
#create feature branch
curl -d "branch=$GITLAB_FEATURE&ref=develop" -X POST "$GITLAB_SERVER/api/v4/projects/$projectId/repository/branches" -H "PRIVATE-TOKEN: $GITLAB_TOKEN" | jq
Repository Activity Checking
The GitLab API also allows you to obtain certain information about files in your repositories, including information like name, size, content, etc. File content is Base64 encoded. This endpoint can be accessed without authentication if the repository is publicly accessible.
Here’s how it works:
`GET /projects/:id/repository/files/:file_path`
curl --header "PRIVATE-TOKEN: <your_access_token>" "https://gitlab.example.com/api/v4/projects/13083/repository/files/app%2Fmodels%2Fkey%2Erb?ref=master"
The above request would give a result like this:
{
"file_name": "key.rb",
"file_path": "app/models/key.rb",
"size": 1476,
"encoding": "base64",
"content": "IyA9PSBTY2hlbWEgSW5mb3...",
"content_sha256": "4c294617b60715c1d218e61164a3abd4808a4284cbc30e6728a01ad9aada4481",
"ref": "master",
"blob_id": "79f7bbd25901e8334750839545a9bd021f0e4c83",
"commit_id": "d5a3ff139356ce33e37e73add446f16869741b50",
"last_commit_id": "570e7b2abdd848b95f2f578043fc23bd6f6fd24d"
}
This can give us all the information we need about the last commit.
Backing Up Data
Having a backup copy of your data is essential for any organization. There are a plethora of reasons why you should back up your data: data loss resulting from unexpected system or server crashes, rogue employees, hacking, or virus attacks are just a few of them. These could cause you to lose your Git repositories, which is why having a solution already in place is so important.
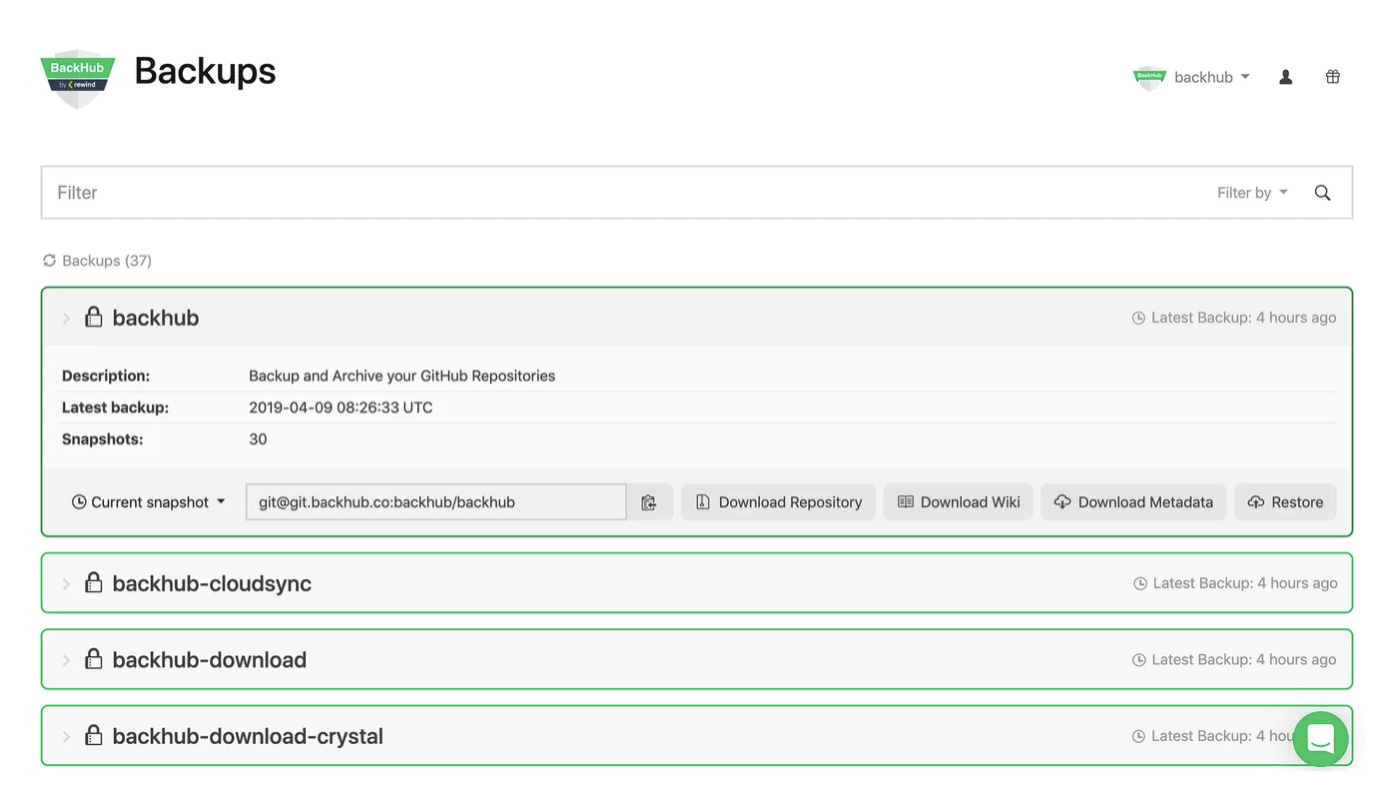
Rewind, formerly known as BackHub, is working on a special feature to improve the reliability of your repositories. With BackHub, you can get a full backup of all your GitLab projects in minutes and have peace of mind as all your backups are updated on a daily basis.
If this sounds great to you, sign up for early access to Rewind Backups for GitLab.
Conclusion
The most significant benefit of managing GitLab repo via an API interface is that it removes silos and provides you with one single source. You will never again have to worry about having to authenticate yourself on different browsers and applications.
Another benefit of using the GitLab API to manage repositories is that it gives you the flexibility to automate many of the mundane tasks you would otherwise have to carry out manually—this gives you a noteworthy edge as a team leader or senior developer.
Furthermore, if your team integrates Jira with GitLab while using the GitLab API for organizing workflows with its repositories, it would provide seamless integration and enhance a smooth workflow.
The bottom line is that every time you integrate GitLab API with the repositories used by your team to automate actions, you reduce the time it takes to complete project administration and other mundane tasks, helping you hit your target at a much faster pace.